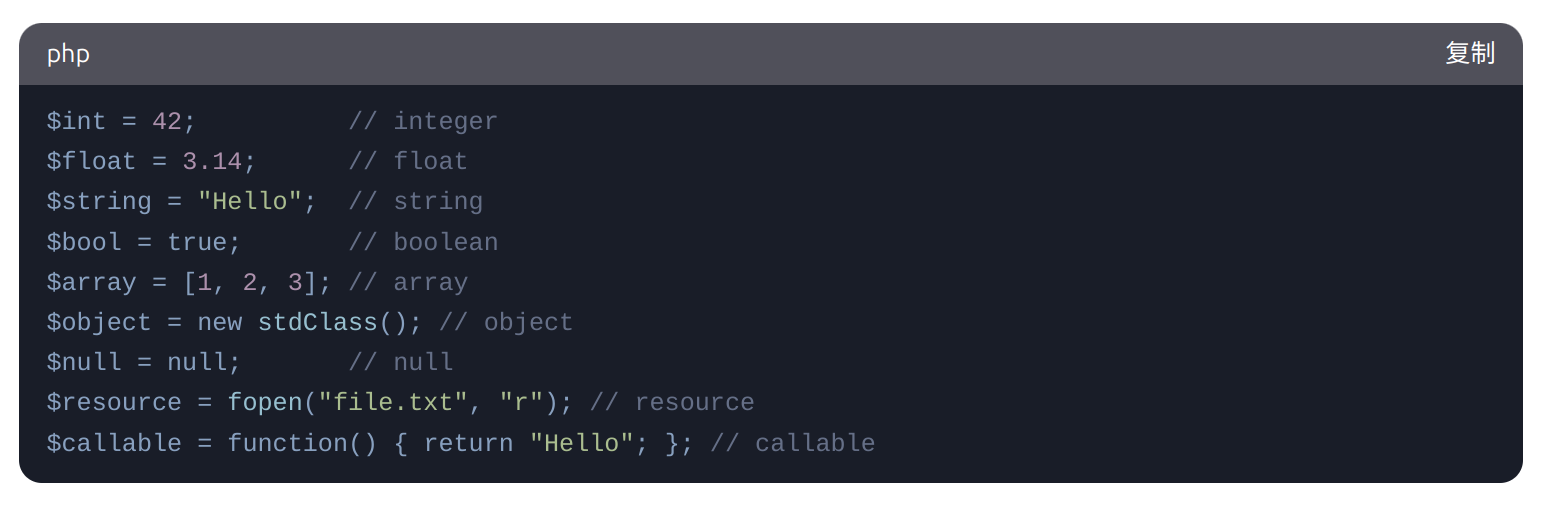
如图上图,
要在代码框右上角实现复制功能,通常需要结合 HTML、CSS 和 JavaScript 来完成。以下是一个简单的实现示例:
1. HTML 结构
<div class="code-container">
<pre><code id="code">
$int = 42; // integer
$float = 3.14; // float
$string = "Hello"; // string
$bool = true; // boolean
$array = [1, 2, 3]; // array
$object = new stdClass(); // object
$null = null; // null
$resource = fopen("file.txt", "r"); // resource
$callable = function() { return "Hello"; }; // callable
</code></pre>
<button class="copy-button" onclick="copyCode()">复制</button>
</div>
运行 HTML
2. CSS 样式
.code-container {
position: relative;
background: #f4f4f4;
padding: 10px;
border: 1px solid #ddd;
border-radius: 4px;
}
pre {
margin: 0;
}
.copy-button {
position: absolute;
top: 10px;
right: 10px;
background: #007bff;
color: white;
border: none;
padding: 5px 10px;
border-radius: 4px;
cursor: pointer;
}
.copy-button:hover {
background: #0056b3;
}
3. JavaScript 功能
function copyCode() {
const code = document.getElementById('code').innerText;
navigator.clipboard.writeText(code).then(() => {
alert('代码已复制到剪贴板!');
}).catch(err => {
console.error('复制失败: ', err);
});
}
4. 完整示例
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>代码复制示例</title>
<style>
.code-container {
position: relative;
background: #f4f4f4;
padding: 10px;
border: 1px solid #ddd;
border-radius: 4px;
}
pre {
margin: 0;
}
.copy-button {
position: absolute;
top: 10px;
right: 10px;
background: #007bff;
color: white;
border: none;
padding: 5px 10px;
border-radius: 4px;
cursor: pointer;
}
.copy-button:hover {
background: #0056b3;
}
</style>
</head>
<body>
<div class="code-container">
<pre><code id="code">
$int = 42; // integer
$float = 3.14; // float
$string = "Hello"; // string
$bool = true; // boolean
$array = [1, 2, 3]; // array
$object = new stdClass(); // object
$null = null; // null
$resource = fopen("file.txt", "r"); // resource
$callable = function() { return "Hello"; }; // callable
</code></pre>
<button class="copy-button" onclick="copyCode()">复制</button>
</div>
<script>
function copyCode() {
const code = document.getElementById('code').innerText;
navigator.clipboard.writeText(code).then(() => {
alert('代码已复制到剪贴板!');
}).catch(err => {
console.error('复制失败: ', err);
});
}
</script>
</body>
</html>
运行 HTML
实现步骤:
HTML:创建一个包含代码和复制按钮的容器。
CSS:设置代码框的样式,并将复制按钮定位在右上角。
JavaScript:使用 navigator.clipboard.writeText 方法将代码复制到剪贴板。
注意事项:
navigator.clipboard.writeText 是现代浏览器支持的 API,如果需要兼容旧版浏览器,可以使用 document.execCommand('copy') 作为备用方案。
如果代码内容较多,可以添加滚动条或限制高度。
通过以上代码,您可以在代码框右上角实现一个简单的复制功能。
评论